It’s no secret that push notifications can help you engage and retain app users. In this tutorial, we'll show you how to integrate with OneSignal (for FREE) in order to leverage push notifications in your Angular app.
OneSignal & Your Browser's Push API
Your web browser's push notification API gives web applications the ability to receive messages from a server whether or not the web app is in the foreground or currently loaded on a user agent. This lets you deliver asynchronous notifications and updates to users who opt-in, resulting in better engagement with timely new content.
What to Know Before Getting Started
Completing this tutorial requires some basic knowledge of Angular. I´m using Angular 12 (the OneSignal NPM package works only with version 11 and above of Angular) and NodeJS version 14.0. If you don't have Angular, create a new Angular project using the Angular CLI.
Guide Overview
- Part 1: Set up Your OneSignal Account
- Web Configuration
- Part 2: Set up Push Notifications in Angular
- Allow Web Push Notifications
- Send Web Push Notifications
Additional Angular Setup Resources:
Part 1: Set Up Your OneSignal Account
To begin, login to your OneSignal account or create a free account. Then, click on the blue button entitled New App/Website to configure your OneSignal account to fit your app or website.
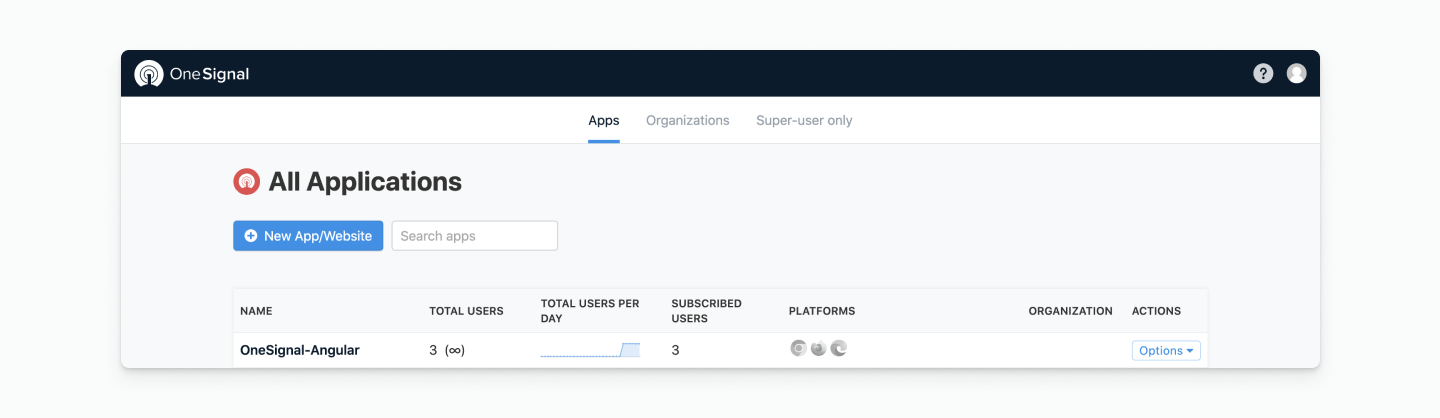
Insert the name of your app or website. Select Web Push as your platform.
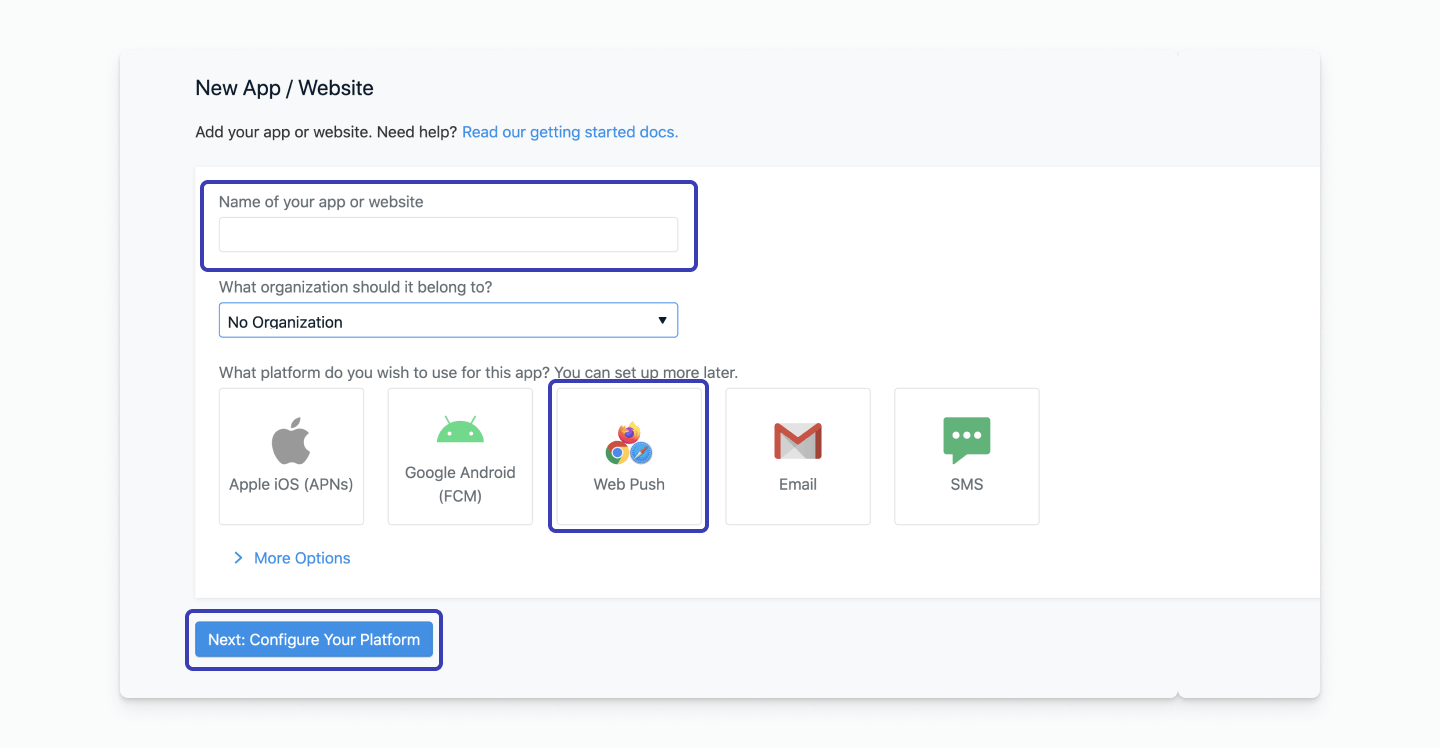
Click on the blue button entitled, Next: Configure Your Platform.
Web Configuration
Under Choose Integration, select the Typical Site option.
In the Site Setup section, enter your chosen web configuration. In my case, the configuration looks like this:
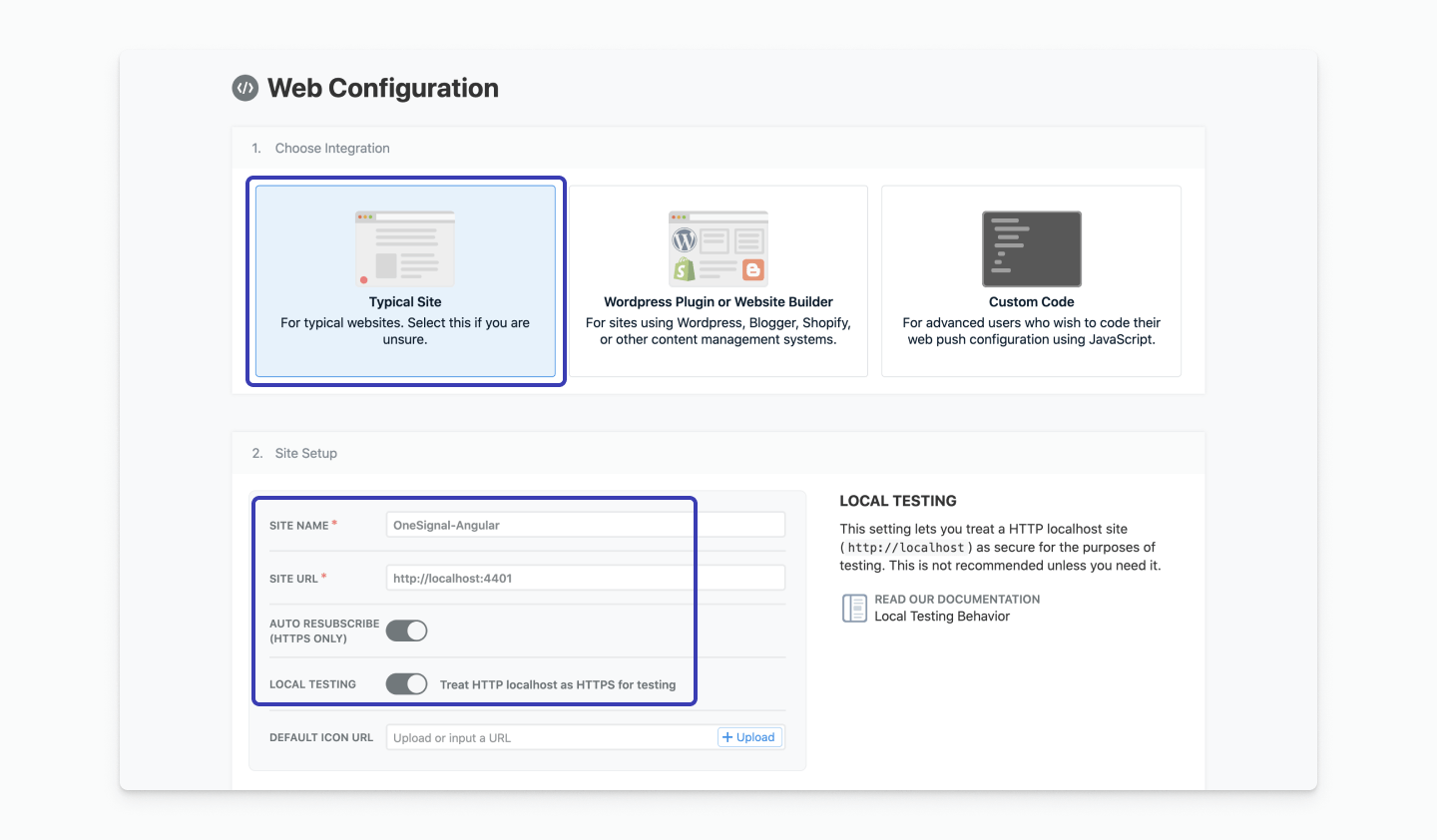
Notice for testing purposes I’m entering my localhost URL (http://localhost:4401). If you are doing the same, make sure you click on the LOCAL TESTING option. This will ensure to treat HTTP localhost as HTTPS for testing.
Under Permission Prompt Setup, you will see three vertical blue dots under the Actions header on the far right side of the screen. Click on the blue dots and select Edit from the drop-down menu.
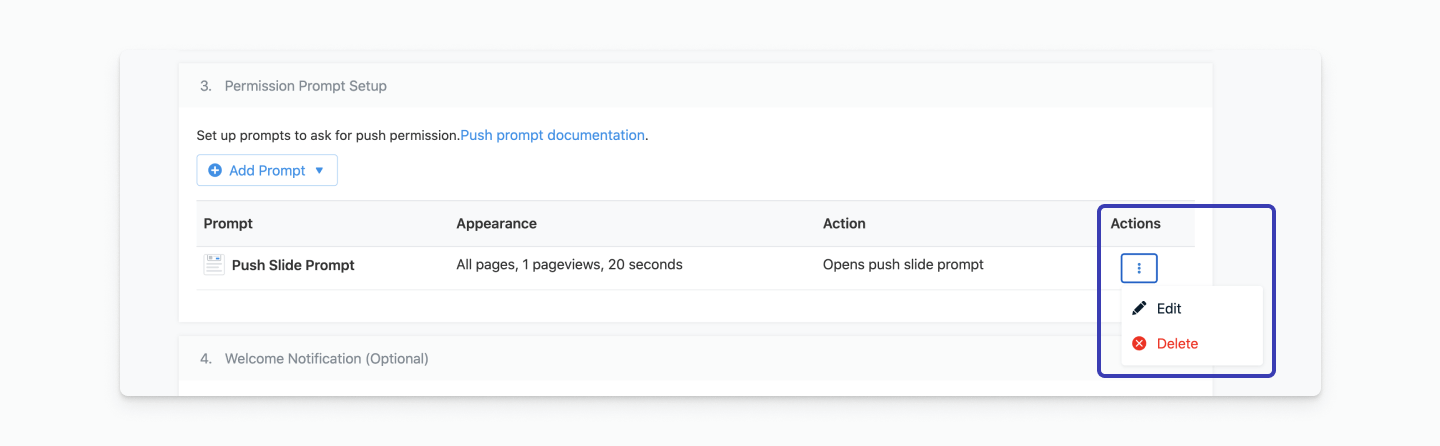
A window will open with the configuration of our push notification Slide Prompt. Confirm that Auto-prompt is enabled (toggled to the right).
Under Show When, you can change the second increment to alter how long your slide prompt will delay after a user visits your page. You can leave it as it is, or you can reduce the seconds so that your prompt appears sooner. Once you've input your chosen delay increment, click the grey Done button located at the bottom right corner of the window.
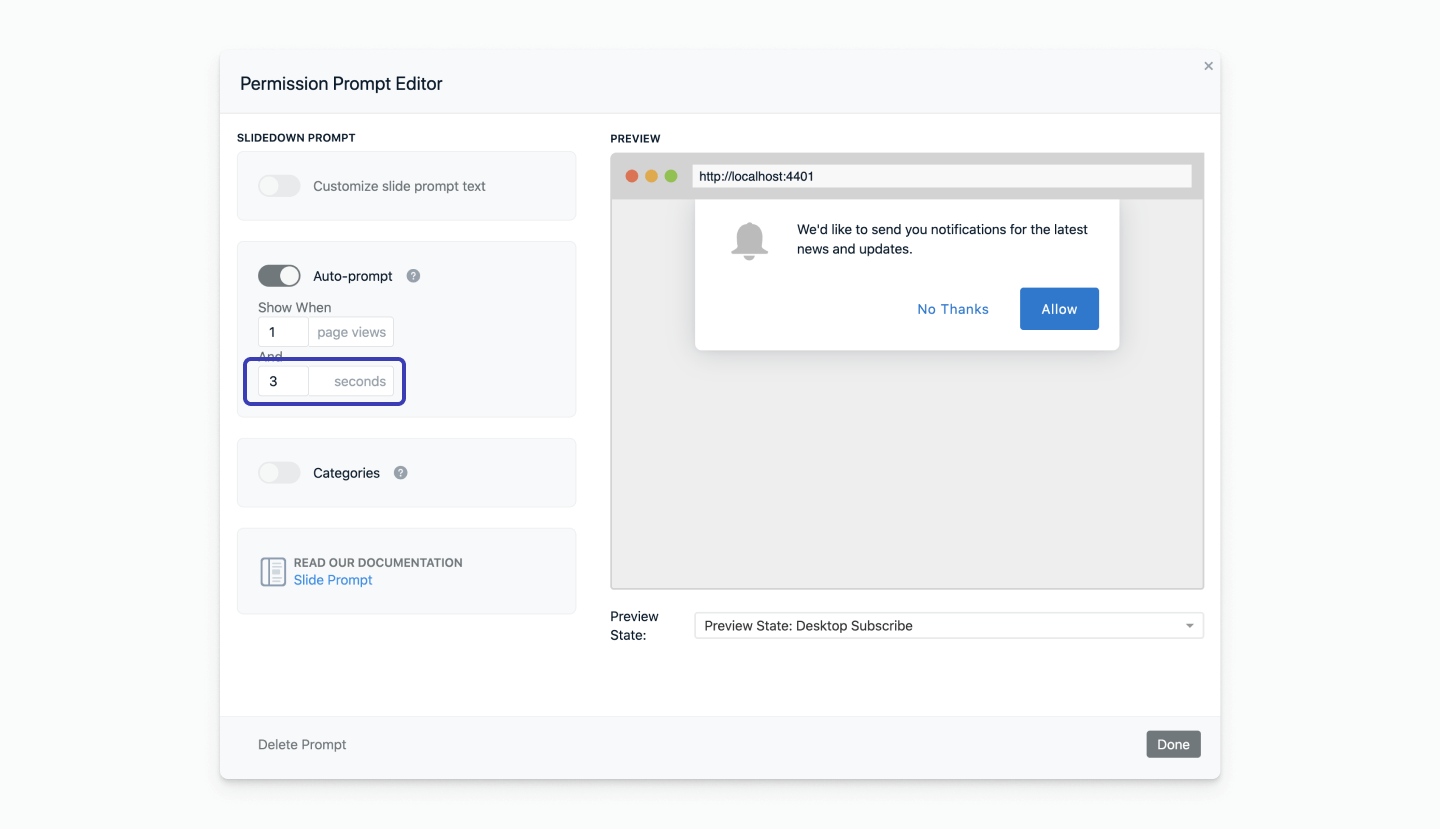
Scroll down to the bottom of the page and click Save to save your auto-prompt selections.
After saving, you will be redirected to a different page with an important step: Downloading the SDK files. Click DOWNLOAD ONESIGNAL SDK FILES and save them on your computer to retrieve later.
Under the section entitled Add Code to Site, look for your appId
. Copy your appId — you will need this later on inside your Angular app.
Part 2: Set up Push Notifications in Angular
Open your terminal in the project folder and run the following command to install the OneSignal NPM package(OneSignal-ngx).
npm i onesignal-ngx
Now, locate the SDK files you downloaded on your computer and insert them inside of the src
folder of your Angular app.
After you have inserted the SDK files into your Angular project, you need to make your Angular component aware of the OneSignal NPM package. To do so, navigate to the component where you want to make use of the OneSignal NPM package. For this example, I’m using the app.component.ts
file because it’s the first component that my app will load.
At the top of your chosen file, import the OneSignalService
from the OneSignal-ngx npm package.
import { OneSignal } from 'onesignal-ngx';
Now, it's time to declare the OneSignal service in your constructor so you can make use of the service to initialize OneSignal in our application. Paste the appId
you previously copied into the space where it says “YOUR-ONESIGNAL-APP-ID”
.
Your app.component.ts
should look like this:
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'angular-example-app';
constructor(private oneSignal: OneSignalService) {
this.oneSignal.init({
appId: "YOUR-ONESIGNAL-APP-ID",
});
}
}
Allow Web Push Notifications
Run the Angular app and visit your website. You should see the following prompt appear after your chosen time delay interval:
Click on the blue Allow button to enable push notifications on your browser.
Send Web Push Notifications
It’s time to send your first web push notification! To do so, login to your OneSignal account and navigate to the Dashboard tab. On the dashboard page, click on the blue button that says New Push.
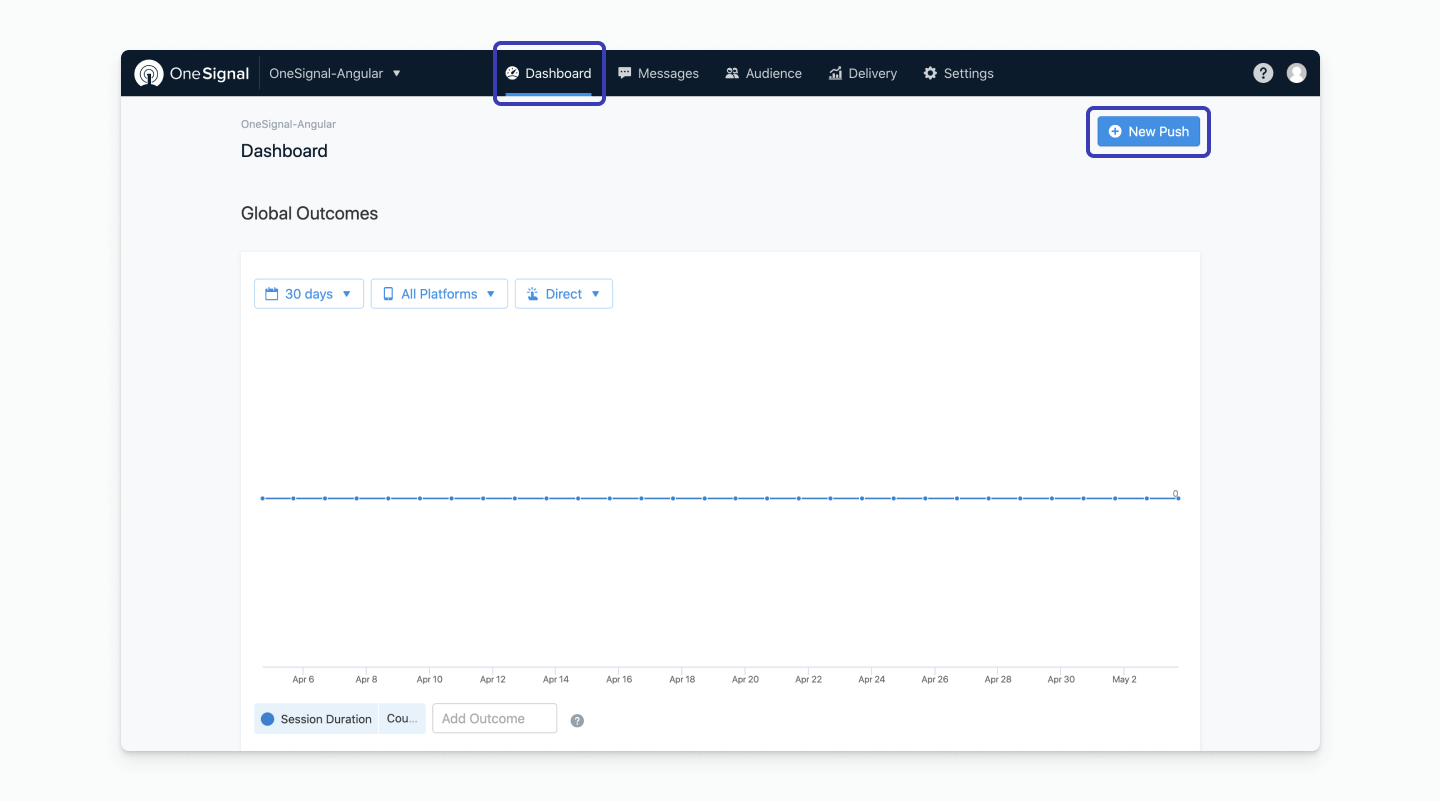
You will be redirected to a new window that will allow you to customize your push notification. Under Audience, make sure that Send to Subscribed Users is selected. Then, create your message by adding your message title, content, and an image. Because this is the first notification your subscribers will receive, you may choose to craft a simple welcome message to confirm that they've been subscribed and reinforce the value that notifications will provide.
Under the Delivery Schedule section, select Immediately and Send to everyone at the same time to send to all your current web push subscribers. If you have just finished setting up your OneSignal account, chances are you're the first and only subscriber. If your app or website is heavily trafficked and other users have already opted in to receive push notifications, you may want to select Send to particular segment(s) to test your message out on a specific audience. When you're ready to send your message, click on the blue Review and Send button at the bottom of the screen.
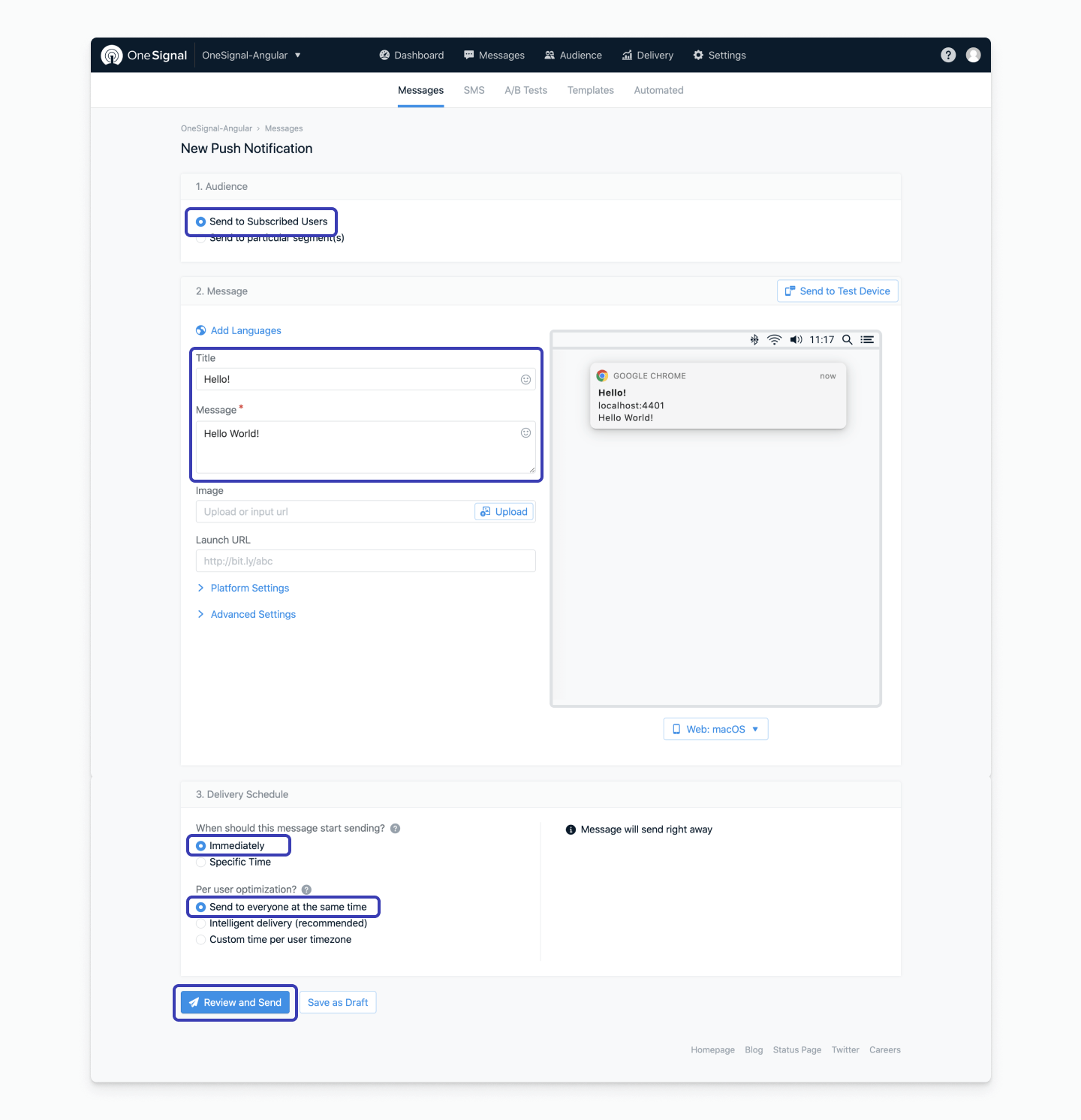
A small popup will appear for you to review your message. Once you are satisfied, click on the blue Send Message button. You should receive a web push notification on your device! 🚀
Now, you can keep expanding your code to make use of different features of the OneSignal SDK across your Angular app. To learn more about the Web Push SDK visit our web push SDK documentation.
Still have questions?
We're here to help. Reach out to us at support@onesignal.com for personalized support.
Stay Connected
Want to share your feedback and test out new features before they're released? Join our developer community to take advantage of these benefits and more.
Join our Developer Community